Introduction
I recently shifted into a team that uses Spring Boot and hence am learning the same. This blog series represents some notes I took along the way.
Spring Boot helps us enable building production ready applications in a short time and provides us some non-functional features like embedded servers, metrics, health checks, etc.
Tools used:
STS 4 (Spring Tool Suite 4) -> https://spring.io/tools
What we will do in this tutorial:
In this part of the blog series, we will setup a simple Spring project and create a REST service that returns a static list of movies and their release dates.
Setting up a basic Spring project
Spring Initializr (https://start.spring.io/) is a site that can quickly help setup a Spring project, capture the required dependencies and package the entire thing into a Maven / Gradle project. This can act as the start up project.
Add the details in the appropriate fields, add “Web” from the dependencies section and click on Generate to generate a zip file.
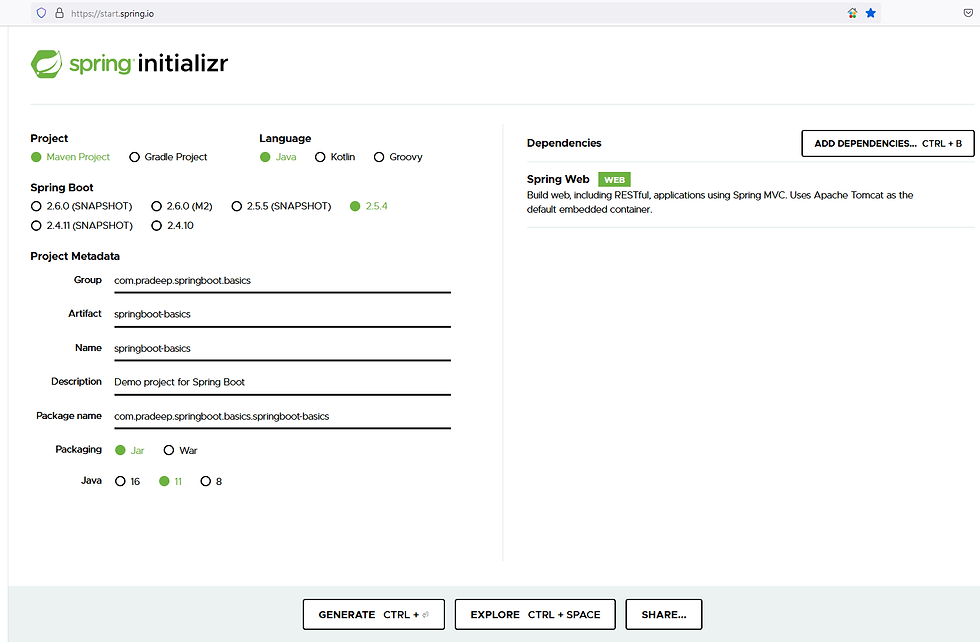
Unzip this and import the same from the STS:

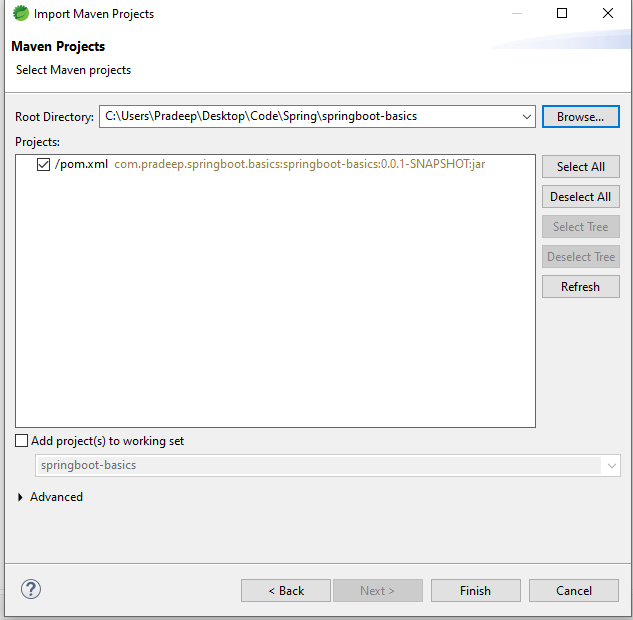
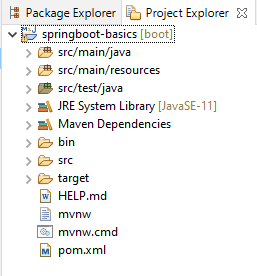
Now, open the main java file and “Run as” a Java application.

You should see some console logs indicating that the application is running on some specific port:
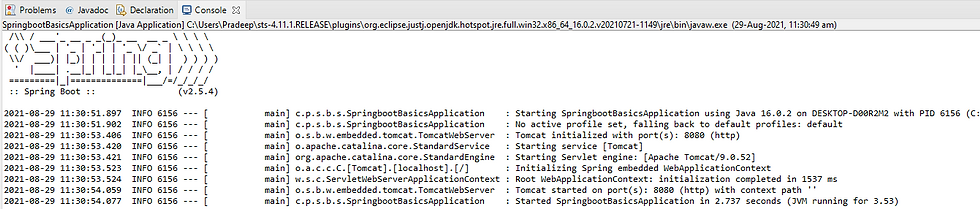
I have uploaded the code to this path:
Setting up a simple REST controller
Now that we have a simple Spring project up and running, let us add a REST controller to it so that it can serve us on a specific path.
Let us create a simple movie info database (a simplified IMDB :P)
First, let us create a class that captures the movie info:

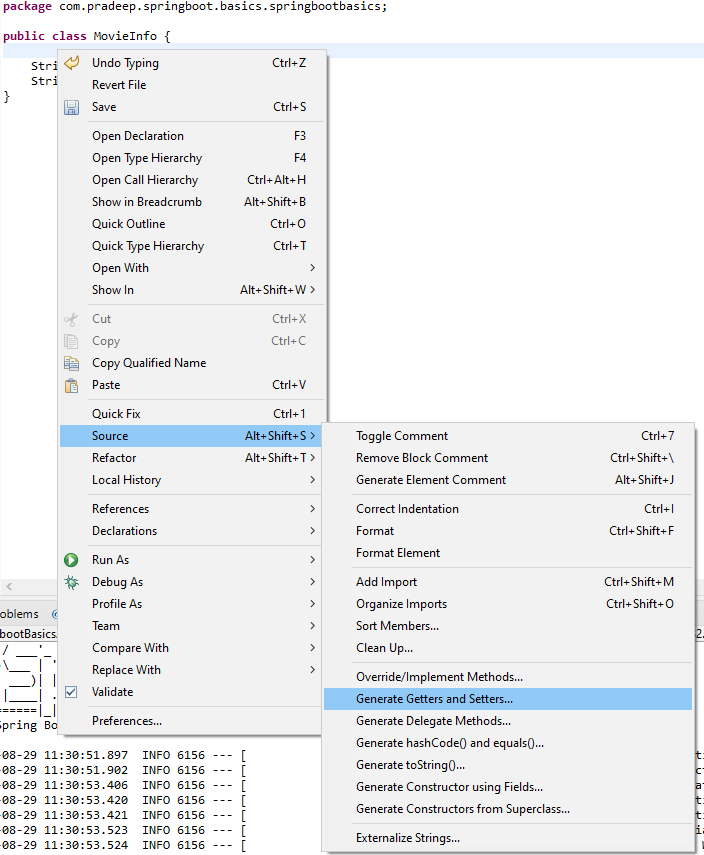
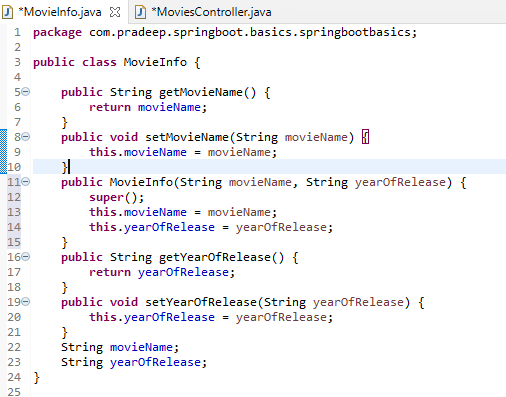
Next step, let us define a REST controller:
Let us define a class called MoviesController and annotate this with an “@RestController”

This has defined a base REST controller but we need to add some methods to perform some actions. So let us add a simple “Get” method that returns a list of movies.
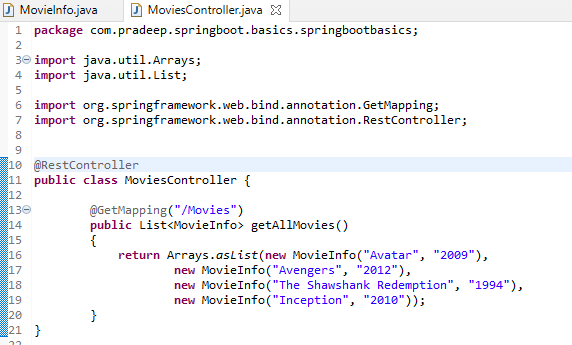
The @GetMapping indicates that the path /Movies should invoke the “getAllMovies” method. This method shall in turn return a static list of some movies and their release years.
Let us run this application now. Run the “MoviesController” as a Java Application. This starts the movies server application. Now, to see if it is running properly, type in http://localhost:8080/Movies into your browser and run it. This shall return the above list:

As you can see, we are also getting the list in the form of a JSON.
Thus, we have created a simple REST controller and added the ability to return a list of movies.
Two important annotations we added here:
Comments