We will see some of the most commonly used docker commands here
For a complete list of the supported commands, please refer to the official documentation.
The Docker run command runs a command on a new container. If the specified docker image doesn’t exist the image cache, docker attempts to download the same and run it.
The Docker run command also runs the default command that is configured when the image is created.
docker run image_name
To override the default command:
docker run image_name command
The command specified here will override the internal default startup command.
We will use busybox to demonstrate this:
C:\Users\Pradeep>docker run busybox ls
Unable to find image ‘busybox:latest’ locally
latest: Pulling from library/busybox
fc1a6b909f82: Pull complete
Digest: sha256:954e1f01e80ce09d0887ff6ea10b13a812cb01932a0781d6b0cc23f743a874fd
Status: Downloaded newer image for busybox:latest
bin
dev
etc
home
proc
root
sys
tmp
usr
var
Some info on busybox:
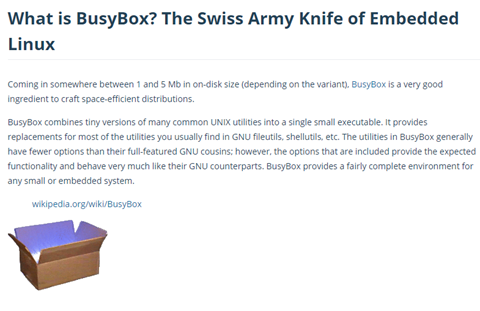
Thus, busybox provides us with a set of command Unix executables. We can run ls, echo, etc. as the startup commands on the busybox image.
Note that we have a couple of flags, -i and -t. Combined, we can use them as
docker run -it imagename initcommand
What this does is, if the init command is to open up a process, for e.g., a unix shell, it does so and maps out command prompt to the process’ stdin, stdout and stderr. Thus, if we want to perform a set of steps on a unix shell, we can do so by launching the busybox with the -it flag:
C:\Users\Pradeep>docker run -it busybox sh
/ # ls
bin dev etc home proc root sys tmp usr var
/ #
This command lists the containers
docker ps
C:\Users\Pradeep>docker ps
CONTAINER ID IMAGE COMMAND CREATED STATUS PORTS NAMES
Since there are no running containers, we don’t see anything listed here.
To list even the stopped containers:
docker ps -a
C:\Users\Pradeep>docker ps -a
CONTAINER ID IMAGE COMMAND CREATED STATUS PORTS NAMES
406ca9d58a4d busybox “sh” 50 seconds ago Exited (0) 30 seconds ago vibrant_dhawan
75547e8e12bd busybox “ls” About a minute ago Exited (0) About a minute ago eager_morse
The docker ps –all does the same too:
docker ps –all
To see the running containers, we need to have container that is going to be alive for more than a few milliseconds. Hence, we can run the following:
C:\Users\Pradeep>docker run busybox ping google.com
PING google.com (216.58.221.206): 56 data bytes
64 bytes from 216.58.221.206: seq=0 ttl=37 time=54.610 ms
64 bytes from 216.58.221.206: seq=1 ttl=37 time=62.091 ms
64 bytes from 216.58.221.206: seq=2 ttl=37 time=62.946 ms
64 bytes from 216.58.221.206: seq=3 ttl=37 time=53.705 ms
…
Now, in a separate command prompt, run the docker ps command:
C:\Users\Pradeep>docker ps
CONTAINER ID IMAGE COMMAND CREATED STATUS PORTS NAMES
fdbc8e636331 busybox “ping google.com” 18 seconds ago Up 16 seconds recursing_nightingale
This shows the details of the currently running container, the command that it is running, etc. The status seen here indicates the difference between a running container and an exited one.
Container Life cycle
When we execute the docker run command, it creates an image and starts it. There are two separate commands that can be run consecutively to achieve the same result:
Docker create and Docker start
The docker create shall create a new container. The created container ID is printed out the std out.
docker create imagefile
C:\Users\Pradeep>docker create hello-world
72dfe62884fcd0f8fc86ae26015b400ede15b50befc2d65970cb034609f07c53
The number printed out here is the container ID
We can see that this container is just created and has not yet run:
C:\Users\Pradeep>docker ps -a
CONTAINER ID IMAGE COMMAND CREATED STATUS PORTS NAMES
72dfe62884fc hello-world “/hello” 5 seconds ago Created youthful_curie
If we want to override the default command for the container, we can do so at this stage:
C:\Users\Pradeep>docker create busybox echo hi
0847903c9977fc17222562e8f8cebb758db5aae0697de4bf1720fcfff110737a
C:\Users\Pradeep>docker ps -a
CONTAINER ID IMAGE COMMAND CREATED STATUS PORTS NAMES
0847903c9977 busybox “echo hi” 7 seconds ago Created quizzical_blackburn
This will create a container whose default command is the “echo hi”.
The docker start command starts the container based on the specified container id
docker start -a containerID
C:\Users\Pradeep>docker start -a 72dfe62884fcd0f8fc86ae26015b400ede15b50befc2d65970cb034609f07c53
Hello from Docker!
This message shows that your installation appears to be working correctly.
…
The -a parameter (a = Attach STDOUT/STDERR and forward signals) is important to display the output from the running container to the command window. Without this, the container shall run and exit with no output printed here.
We have to note that we cannot provide an overriding default command here. Whatever default command that the container was created with, using the docker create or docker run, shall be executed when we run the docker start on that container. Earlier, we created the:
C:\Users\Pradeep>docker create busybox echo hi
e59e409c174bc061441f71a66da99d7a5fc6b66ce414b184c02671615fdeabb9
This generated a container with ID e59e409c174bc061441f71a66da99d7a5fc6b66ce414b184c02671615fdeabb9
If we run the same now:
C:\Users\Pradeep>docker start -a e59e409c174b
hi
Note that we need not specify the full image ID. An arbitrary number of initial digits is sufficient for docker to understand which container we are talking about.
To stop any running container, we can use the docker stop command
Using the docker ps, we can see the running containers:
C:\Users\Pradeep>docker ps
CONTAINER ID IMAGE COMMAND CREATED STATUS PORTS NAMES
4bc0ad87a5b5 busybox “ping google.com” 3 seconds ago Up 2 seconds fervent_gauss
To stop this, we can use the docker stop command
C:\Users\Pradeep>docker stop 4bc0ad87a5b5
4bc0ad87a5b5
C:\Users\Pradeep>docker ps
CONTAINER ID IMAGE COMMAND CREATED STATUS PORTS NAMES
The docker stop command has a default grace period of 10 seconds. Thus, once the command to stop a container is issued, it waits for 10 seconds before killing it. This can be changed with the -t attribute.
The docker stop command sends a SIGTERM to the primary process in the container once run. This indicates to the container that it needs to stop what it’s doing and close up shop. This means that the container has some time to do a graceful exit. After 10 seconds (default value), a SIGKILL is sent to the container. If we need to stop a container gracefully, where in some running process needs to deregister itself, etc., we can use this docker stop command with an appropriate time value.
If we need to stop an unresponsive container or do an immediate termination, we can use the docker kill command instead or specify the time param as 0.
C:\Users\Pradeep>docker ps
CONTAINER ID IMAGE COMMAND CREATED STATUS PORTS NAMES
7b1da29930ed busybox “ping google.com” 4 seconds ago Up 3 seconds festive_hermann
C:\Users\Pradeep>docker stop -t 0 7b1da29930ed
7b1da29930ed
C:\Users\Pradeep>docker ps
CONTAINER ID IMAGE COMMAND CREATED STATUS PORTS NAMES
The docker kill command sends a SIGKILL signal to the container’s primary process and kills it immediately.
C:\Users\Pradeep>docker ps
CONTAINER ID IMAGE COMMAND CREATED STATUS PORTS NAMES
ff35cecf21db busybox “ping google.com” 6 seconds ago Up 4 seconds elated_zhukovsky
4a57fe349f22 busybox “ping google.com” 7 minutes ago Up 7 minutes laughing_galois
C:\Users\Pradeep>docker kill ff35cecf21db
ff35cecf21db
C:\Users\Pradeep>docker ps
CONTAINER ID IMAGE COMMAND CREATED STATUS PORTS NAMES
4a57fe349f22 busybox “ping google.com” 8 minutes ago Up 8 minutes laughing_galois
This can be used to kill unresponsive containers too.
The docker system can be used with a few commands to manage the docker system:
docker system df Show docker disk usage
docker system events Get real time events from the server
docker system info Display system-wide information
docker system prune Remove unused data
The prune command shall remove the stopped containers, the image caches that are not currently in use, etc.
C:\Users\Pradeep>docker system prune
WARNING! This will remove:
– all stopped containers
– all networks not used by at least one container
– all dangling images
– all dangling build cache
Are you sure you want to continue? [y/N] y
Deleted Containers:
8a8a293cea1df9d7631e5e7b9ec4b51595e669f3f9290b5e1c2de8181028ffbd
e6d76d940170d1c7149eb9fc0e8bd755c7eb170e6ff650b34565ce79974a30f3
ae15b5ff15ecd9ecd5b66ab39dfaa420e87bae376e89b40d52b561e00f63c25d
725472dee9e7060cadeb831d2887cc1a1727b6c3af203a06655154a13daeba51
Deleted Images:
untagged: pradeep87blore/simplepythonwebserver@sha256:49f6b6e3cc22a2b1e93ab85d0536cccb64b237a2bce678d4e7a89cc4af5c978a
deleted: sha256:3e982451d5efe97ded20296282aceeecbc5b3a413629cbced5f8b38d4ea6414e
deleted: sha256:7e2acd2807e38ad0e07ffea639d880aa6d252a1e2991278574429c982446a516
deleted: sha256:ecd6df333189dc917f68135a59e1a2e93ccce168fe19bcbc562ad01be467042e
deleted: sha256:f81af53dba01d15d445c5547abfe5a38741604d6cef090a8e2d27a57f4664356
Total reclaimed space: 54.6MB
docker system prune -a removes even the unused images, not just the dangling ones. This means that docker will have to redownload the same the next time we run such an image.
C:\Users\Pradeep>docker system prune -a
WARNING! This will remove:
– all stopped containers
– all networks not used by at least one container
– all images without at least one container associated to them
– all build cache
Are you sure you want to continue? [y/N] y
Deleted Containers:
56bb228d45c13db3bdfa8b835fd0ff2a545cf38a3e5daa3b9ca20543e4eaec12
Deleted Images:
untagged: docker4w/nsenter-dockerd:latest
deleted: sha256:2f1c802f322feffe042c978b11540ceea34d019df7475fcb1dd4d2d67c0538ba
deleted: sha256:f0c903ccb59e79a36a0bebae70d1de4ccc7e63588effe9108b04c04d8d96554b
untagged: hello-world:latest
untagged: hello-world@sha256:2557e3c07ed1e38f26e389462d03ed943586f744621577a99efb77324b0fe535
deleted: sha256:fce289e99eb9bca977dae136fbe2a82b6b7d4c372474c9235adc1741675f587e
deleted: sha256:af0b15c8625bb1938f1d7b17081031f649fd14e6b233688eea3c5483994a66a3
untagged: pradeep87blore/simplepythonwebserver:latest
untagged: pradeep87blore/simplepythonwebserver@sha256:d12d15560063b188f7576ad3067bff37901d774f1618b65929a7dd4644af8c9b
deleted: sha256:dea0123f5f9b58c94dea61a774abc9188790e788792b17f9968b4dd79cd7cdea
deleted: sha256:edcab208e715c0632c051b19917232274f371648209c243f764215e2607f8299
deleted: sha256:c62c6743ae2374322f99b76a8eee5f537ee3022df447a009e3c047ff974e5ed7
deleted: sha256:491708fe8a289e6db35517e2f68a54586ca760fa7c898ec973464e9109cb525b
deleted: sha256:8d69ea2e8d573587132cb1ecd13390e0e92ab648ffbdd0e1c5c453ddf4ae17a4
deleted: sha256:984db1f67f3bb2f04ba7728d9da643c0776d29ac278448acd9c8d88b5a839f68
deleted: sha256:b5ba0706254e399b33a7c4dbb96b3eae68e1631816da21e3269f3c32fa004cdd
deleted: sha256:59fea59c609989966f1b789e8463d950ecfa4ea50d2a340828bf697d8bdefa2f
deleted: sha256:342508d0c2e1ca5b8c3bd60f0e197660abb58d09bb5a22e9218dd28fd4e408ad
deleted: sha256:c631cfee89118eaefc3266b1d3d877ba31b82eecbbb23a24d276ceeb13c01a13
untagged: busybox:latest
untagged: busybox@sha256:954e1f01e80ce09d0887ff6ea10b13a812cb01932a0781d6b0cc23f743a874fd
deleted: sha256:af2f74c517aac1d26793a6ed05ff45b299a037e1a9eefeae5eacda133e70a825
deleted: sha256:0b97b1c81a3200e9eeb87f17a5d25a50791a16fa08fc41eb94ad15f26516ccea
untagged: alpine:latest
untagged: alpine@sha256:644fcb1a676b5165371437feaa922943aaf7afcfa8bfee4472f6860aad1ef2a0
deleted: sha256:5cb3aa00f89934411ffba5c063a9bc98ace875d8f92e77d0029543d9f2ef4ad0
deleted: sha256:bcf2f368fe234217249e00ad9d762d8f1a3156d60c442ed92079fa5b120634a1
Total reclaimed space: 61.52MB
C:\Users\Pradeep>docker run busybox echo hi
Unable to find image ‘busybox:latest’ locally
latest: Pulling from library/busybox
fc1a6b909f82: Pull complete
Digest: sha256:954e1f01e80ce09d0887ff6ea10b13a812cb01932a0781d6b0cc23f743a874fd
Status: Downloaded newer image for busybox:latest
hi
As it can be seen here, using the -a option deleted even the unused busybox image and docker redownloaded the same the next time we ran it.
This command shall provide us with the disk usage info of docker:
C:\Users\Pradeep>docker system df
TYPE TOTAL ACTIVE SIZE RECLAIMABLE
Images 2 2 1.201MB 0B (0%)
Containers 2 0 0B 0B
Local Volumes 0 0 0B 0B
Build Cache 0 0 0B 0B
The docker logs command provide the logs output by a container till the point at which this command was run. There are various attributes to filter out the logs based on the timestamp, count, etc. Check the documentation for more info.
C:\Users\Pradeep>docker ps -a
CONTAINER ID IMAGE COMMAND CREATED STATUS PORTS NAMES
2b0cfb9d7113 busybox “echo hi” 59 seconds ago Exited (0) 57 seconds ago peaceful_payne
b1897644c1b0 hello-world “/hello” About a minute ago Exited (0) About a minute ago sad_burnell
C:\Users\Pradeep>docker logs 2b0cfb9d7113
hi
Note that the logs command doesn’t run the container again. It just prints out the logs that were generated when the container ran earlier. This can also be used in case we ran the docker start with out the -a option but would like to know what the output was earlier.
Consider a situation where we want to run a second command on an already running container. To do this, we can use the docker exec.
First, let us get the busybox container running by letting it ping google.com:
C:\Users\Pradeep>docker run busybox ping google.com
PING google.com (216.58.221.206): 56 data bytes
64 bytes from 216.58.221.206: seq=0 ttl=37 time=57.755 ms
64 bytes from 216.58.221.206: seq=1 ttl=37 time=56.541 ms
64 bytes from 216.58.221.206: seq=2 ttl=37 time=56.389 ms
64 bytes from 216.58.221.206: seq=3 ttl=37 time=56.030 ms
As we know, this will keep running till we kill it manually
C:\Users\Pradeep>docker ps
CONTAINER ID IMAGE COMMAND CREATED STATUS PORTS NAMES
4a57fe349f22 busybox “ping google.com” 19 seconds ago Up 17 seconds laughing_galois
Assume we want to run the echo command on this container. We can do so as follows:
C:\Users\Pradeep>docker exec -it 4a57fe349f22 echo hi
hi
Thus, we were able to send a second command to the already running container. This example shown here might be a trivial one and a very simple use case but the actual use cases for this command might be much more complicated.
Note that the docker ps still shows the initial command and not the one supplied with the exec
C:\Users\Pradeep>docker ps
CONTAINER ID IMAGE COMMAND CREATED STATUS PORTS NAMES
4a57fe349f22 busybox “ping google.com” About a minute ago Up About a minute laughing_galois
Note that we don’t need the -it flags (-i and -t together) in case we don’t need to connect to any process that we are starting off in the container with the second command:
C:\Users\Pradeep>docker exec 4a57fe349f22 echo hi
hi
There are situations where we will need to be able to access the process that was started off with the exec command. That is when the -it param comes into play. When a container is running, we can use the -it flags to gain access to the stdin, stdout and stderr of the process and map this to our command prompt.
A simple example will be, with the busybox container running, we might need to start the vi on the same. If we use the command exec without the -it flag, the vi opens but we will not have any way to access the same:
C:\Users\Pradeep>docker exec 4a57fe349f22 vi
~
~
~
~
~
~
~
~
~
~
~
~
~
~
~
~
~
~
~
~
~
~
vi: can’t read user input
C:\Users\Pradeep>^[[30;120R
Now, if we use the same with the -it flag:
C:\Users\Pradeep>docker exec -it 4a57fe349f22 vi
Hi World
~ ~ ~ ~ ~ ~ ~ ~ ~ ~ ~ ~ ~ ~ ~ ~ ~ ~ ~ ~ ~ ~ ~ ~ ~ ~ ~ ~ I No file 1/1 100%
The cmd prompt gets mapped to the vi stdin, stdout and stderr and thus, we will be allowed to use this running process from the command prompt.
Another simple use is to start the unix shell. We can use the -it flags on a running container and use the sh command to start the unix shell:
C:\Users\Pradeep>docker exec -it 4a57fe349f22 sh
/ # ls
bin dev etc home proc root sys tmp usr var
/ #
Note that we are keeping this container from ending as long as we have such a mapping open.
Doing a Ctrl C or Ctrl D will bring us out of the mapping and might allow the container to end
C:\Users\Pradeep>docker exec -it 4a57fe349f22 sh
/ # ls
bin dev etc home proc root sys tmp usr var
/ # ^C
/ #
C:\Users\Pradeep>docker ps
CONTAINER ID IMAGE COMMAND CREATED STATUS PORTS NAMES
4a57fe349f22 busybox “ping google.com” 6 minutes ago Up 6 minutes laughing_galois
Just another example with the Ubuntu image and the bash shell:
C:\Users\Pradeep>docker run -it ubuntu sh
Unable to find image ‘ubuntu:latest’ locally
latest: Pulling from library/ubuntu
898c46f3b1a1: Pull complete
63366dfa0a50: Pull complete
041d4cd74a92: Pull complete
6e1bee0f8701: Pull complete
Digest: sha256:017eef0b616011647b269b5c65826e2e2ebddbe5d1f8c1e56b3599fb14fabec8
Status: Downloaded newer image for ubuntu:latest
# ls
bin boot dev etc home lib lib64 media mnt opt proc root run sbin srv sys tmp usr var
#
Comments